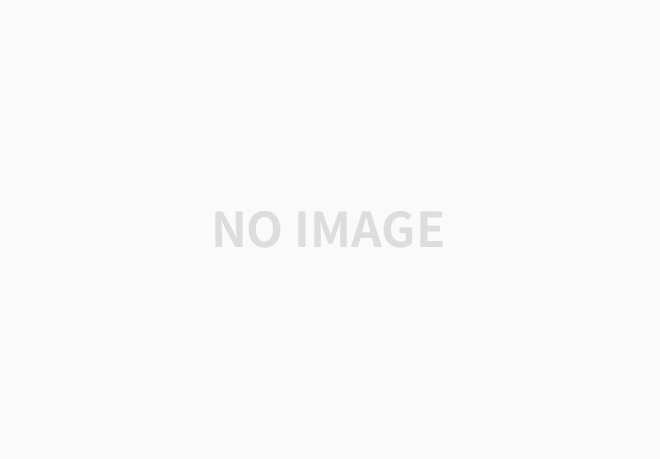
Props (์์ฑ)
- ๋ถ๋ชจ -> ์์ ์ปดํฌ๋ํธ๋ก ๋ฐ์ดํฐ๋ฅผ ์ ๋ฌํ ๋ ์ฌ์ฉํ๋ค.
- ์ฝ๊ธฐ ์ ์ฉ์ด๋ฉฐ, ์์ ์ปดํฌ๋ํธ์์ ์์ ํ ์ ์๋ค.
// ๋ถ๋ชจ ์ปดํฌ๋ํธ
import React from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = () => {
const data = "์ ๋ฌํ ๋ฐ์ดํฐ";
return <ChildComponent data={data} />;
};
// ์์ ์ปดํฌ๋ํธ
import React from 'react';
const ChildComponent = (props) => {
return <div>{props.data}</div>;
};
State (์ํ)
- ์ปดํฌ๋ํธ ๋ด๋ถ์์ ๊ด๋ฆฌ ํ๋ ๋ฐ์ดํฐ์ด๋ค.
- ์ปดํฌ๋ํธ์ ์ํ๊ฐ ๋ณํํ ๋๋ง๋ค React๋ ์๋์ผ๋ก ๋๋๋งํ๋ค.
- State์ ์ด๊ธฐํ๋ ์ปดํฌ๋ํธ์ constructor์์ ์ํํ๋ค.
- State ๋ณ๊ฒฝ ์, State๋ฅผ ์ง์ ์์ ํด์๋ ์๋๊ณ , SetState ๋ฉ์๋๋ฅผ ์ฌ์ฉํ์ฌ ๋ณ๊ฒฝ์ ๋ฐ์ํ๋ค.
- ํด๋์คํ ์ปดํฌ๋ํธ์์๋ this.State๋ก State์ ์ ๊ทผํ๊ณ , ํจ์ํ ์ปดํฌ๋ํธ์์๋ useState ํ ์ ์ฌ์ฉํ๋ค.
// ํด๋์คํ ์ปดํฌ๋ํธ์์์ state
import React, { Component } from 'react';
class ExampleComponent extends Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
incrementCount = () => {
this.setState({ count: this.state.count + 1 });
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.incrementCount}>Increment</button>
</div>
);
}
}
// ํจ์ํ ์ปดํฌ๋ํธ์์์ state
import React, { useState } from 'react';
const ExampleComponent = () => {
const [count, setCount] = useState(0);
const incrementCount = () => {
setCount(count + 1);
}
return (
<div>
<p>Count: {count}</p>
<button onClick={incrementCount}>Increment</button>
</div>
);
}
๋ฐ์ํ
'Frontend > React' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
React | ์๋ช ์ฃผ๊ธฐ ๋ฉ์๋(Lifecyle Methods) (0) | 2023.11.28 |
---|---|
React | ๊ฐ์ฒด ํ์ State ์ ๋ฐ์ดํธ (0) | 2023.11.27 |
React | ๋ฐฐ์ด ํ์ State ์ ๋ฐ์ดํธ (1) | 2023.11.24 |
React | ๋ณ์ vs State (2) | 2023.11.23 |
React | event (์ด๋ฒคํธ) ์ ๋ํด์ (0) | 2023.11.18 |